We will construct a short piece of tube introducing the function pnf_ube_pnf_v() from pnf_cylinder.scad.
Take a look at the following listing and the generated output in the picture below.
use <inc/pnf/pnf_cylinder.scad>
fn = 128;
height = 100;
outer_radius = 30;
wall_thickness = 4;
v = tube_pnf_v(
r = outer_radius,
h = height,
wt = wall_thickness, nz = 1, fn = fn);
polyhedron(v[0], v[1], 4);
So, what’s so special about this? Why shouldn’t you use the following?
linear_extrude(height=height)
difference()
{
circle(r=outer_radius, $fn=fn);
circle(r=outer_radius-wall_thickness, $fn=fn);
}
It would produce the same output:
Well, the difference starts, when you want something different. With the usual OpenSCAD functionality it is easy to cut away parts or create more complex structures by putting several simple objects together, but you are always limited to what you get from the basic primitives. You can apply rotate_extrude() on a difference() of 2 circle()s and cut out 1/4 of it to make a 90° bent tube or you can apply rotate_extrude() on a polygon() fed with a bent line created with a for-assignment to create a tube that is narrower in the middle, but you can’t combine both mutations in one object.
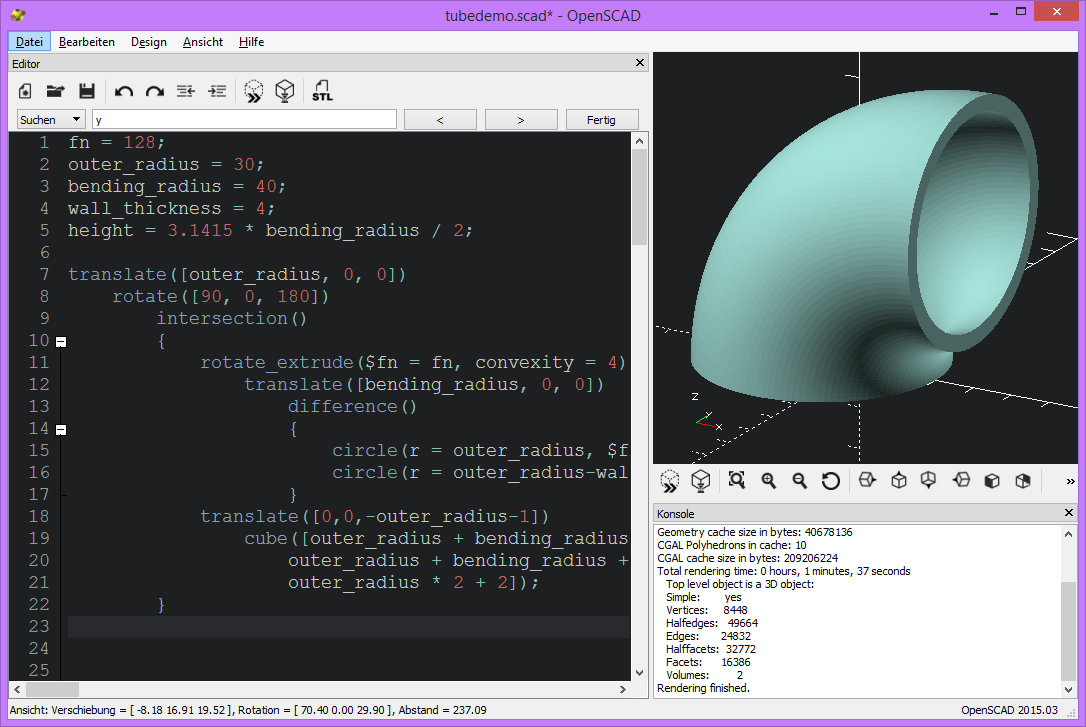
fn = 128;
outer_radius = 30;
bending_radius = 40;
wall_thickness = 4;
height = 3.1415 * bending_radius / 2;
translate([outer_radius, 0, 0])
rotate([90, 0, 180])
intersection()
{
rotate_extrude($fn = fn, convexity = 4)
translate([bending_radius, 0, 0])
difference()
{
circle(r = outer_radius, $fn = fn);
circle(r = outer_radius-wall_thickness, $fn = fn);
}
translate([0,0,-outer_radius-1])
cube([outer_radius + bending_radius + 1,
outer_radius + bending_radius + 1,
outer_radius * 2 + 2]);
}
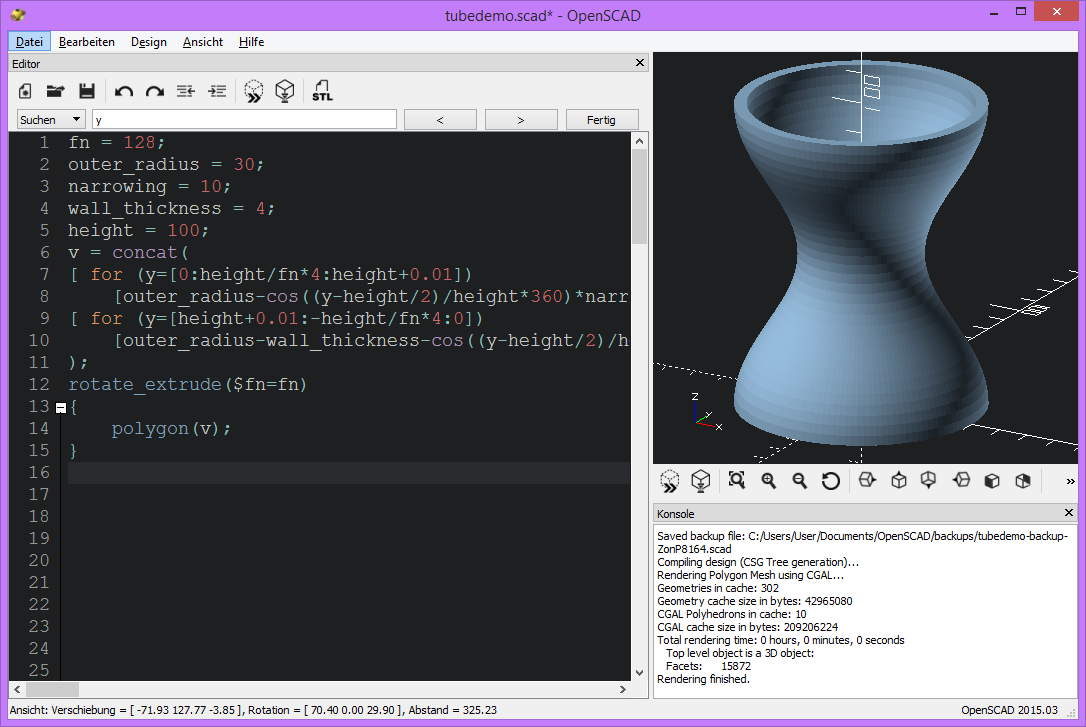
fn = 128;
outer_radius = 30;
narrowing = 10;
wall_thickness = 4;
height = 100;
v = concat(
[ for (y=[0:height/fn*4:height+0.01])
[outer_radius-cos((y-height/2)/height*360)*narrowing,y]],
[ for (y=[height+0.01:-height/fn*4:0])
[outer_radius-wall_thickness-cos((y-height/2)/height*360)*narrowing,y] ]
);
rotate_extrude($fn=fn)
{
polygon(v);
}
So how about a bent tube with a narrower middle? Then let’s take a look at the less simple example!